티스토리 뷰
드디어 마지막 Redux Toolkit이다아아
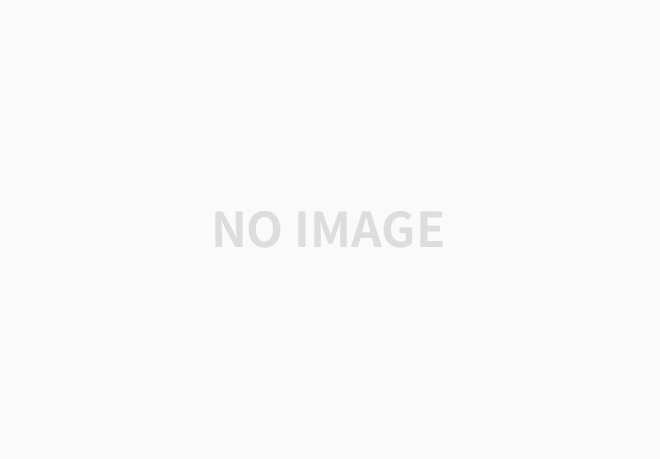
Redux Toolkit
Redux Toolkit은
Redux를 좀 더 간편하게 만들 수 있는 도구이다.
으음.. react로 비유하자면 hook 같은 느낌!
life Cycle을 이해하고 쓴다면 코드를 엄청 줄일 수 있는 강력한 도구!
설치
일단 터미널을 통하여
npx create-react-app my-app --template redux
터미널에 해당 명령어를 입력하여
redux를 포함한 react 프로젝트를 생성한다.
npm install @reduxjs/toolkit
이번에는 toolkit두 설치해주자!
코드
이번 코드는 바로 전 Redux-react에서의 코드를
toolkit으로 얼마나 효율적으로 바꿀 수 있는지가 핵심임으로
저번 코드에서 가져와서 할 거다
Redux by react
목차 당신에게 Redux는 필요 없을지도 모릅니다. 이 글은 Dan Abramov의 You Might Not Need Redux를 번역한 글입니다. medium.com Redux 공식 홈페이지에 올라와 있는 글 중 하나이다. 구글링을 하면서 리덕스에.
sirong.tistory.com
안 보고 왔으면 보고 오자.
redux를 이해하지 않고 toolkit을 사용하는 것은 위험하다
before
//Store.js
import { createStore } from "redux";
const PLUS = "PLUS";
const MINUS = "MINUS";
const actionPlusOne = (number) => {
return {
type: PLUS,
number
};
};
const actionMinusOne = (number) => {
return {
type: MINUS,
number
};
};
function Store (number = 0, action) {
switch(action.type){
case PLUS:
return number +1;
case MINUS:
return number -1;
default:
return number;
}
}
const countStore = createStore(Store);
export default Store;
export const actions = {
actionPlusOne,
actionMinusOne
};
after
//Store.js
import { createStore } from "redux";
import { createAction, createReducer } from "@reduxjs/toolkit";
const actionPlusOne = createAction("PLUS");
const actionMinusOne = createAction("MINUS");
const Store = createReducer(0, {
[actionPlusOne]: (state, action) => {
return action.payload+1;
},
[actionMinusOne]: (state, action) => {
return action.payload-1;
},
})
const countStore = createStore(Store);
export const actions = {
actionPlusOne,
actionMinusOne
};
export default countStore;
해석
redux Toolkit을 쓴 부분은
createAction
import { createStore } from "redux";
import { createAction } from "@reduxjs/toolkit";
const actionPlusOne = createAction("PLUS");
const actionMinusOne = createAction("MINUS");
// const PLUS = "PLUS";
// const MINUS = "MINUS";
// const actionPlusOne = (number) => {
// return {
// type: PLUS,
// number
// };
// };
// const actionMinusOne = (number) => {
// return {
// type: MINUS,
// number
// };
// };
const Store = (number = 0, action) => {
switch(action.type){
case actionPlusOne.type:
// case PLUS:
return action.payload +1;
// return action.number +1;
case actionMinusOne.type:
// case MINUS:
return action.payload -1;
// return action.number -1;
default:
return number;
}
};
const countStore = createStore(Store);
export const actions = {
actionPlusOne,
actionMinusOne
};
export default countStore;
createAction은 말 그대로
Redux 저장소에서 action을 만들어주는 함수이다.
예로 들어
const PLUS = "PLUS";
const actionPlusOne = (number) => {
return {
type: PLUS,
number
};
};
action을 정의하기 위해, 이렇게 코드를 짜야했던걸
const actionPlusOne = createAction("PLUS");
이렇게 한 줄로 줄일 수 있다.
이에 따라, 저장소에서 변경을 좀 줘야 하는데
const Store = (number = 0, action) => {
switch(action.type){
case PLUS:
return action.number +1;
case MINUS:
return action.number -1;
default:
return number;
}
};
해당 저장소를
const Store = (number = 0, action) => {
switch(action.type){
case actionPlusOne.type:
return action.payload +1;
case actionMinusOne.type:
return action.payload -1;
default:
return number;
}
};
createAction를 담은 변수의 type를 switch.case로 담고
number로 명시하여 받아왔던 데이터는
playload로 통일되어 가져와진다.
데이터가 여러 개일 경우,
payload: {
number: 3,
state: 'OK'
}
이렇게 payload라는 object안에 묶인다.
createReducer
import { createStore } from "redux";
import { createAction, createReducer } from "@reduxjs/toolkit";
const actionPlusOne = createAction("PLUS");
const actionMinusOne = createAction("MINUS");
// const Store = (number = 0, action) => {
// switch(action.type){
// case actionPlusOne.type:
// // case PLUS:
// return action.payload +1;
// // return action.number +1;
// case actionMinusOne.type:
// // case MINUS:
// return action.payload -1;
// // return action.number -1;
// default:
// return number;
// }
// };
const Store = createReducer(0, {
[actionPlusOne]: (state, action) => {
return action.payload+1;
},
[actionMinusOne]: (state, action) => {
return action.payload-1;
},
})
const countStore = createStore(Store);
export const actions = {
actionPlusOne,
actionMinusOne
};
export default countStore;
createReducer은 reducer Store를
더 깔끔하게 만들어주는 함수이다.
const Store = (number = 0, action) => {
switch(action.type){
case actionPlusOne.type:
return action.payload +1;
case actionMinusOne.type:
return action.payload -1;
default:
return number;
}
};
이 부분을
const Store = createReducer(0, {
[actionPlusOne]: (state, action) => { return action.payload+1;},
[actionMinusOne]: (state, action) => { return action.payload-1;},
})
이렇게 대체할 수 있다.
일단 장점으로써, switch를 안 써도 된다는 것,
또한 추후에 다른 포스팅으로 안내할 것인데,
Redux는 data를 변경했을 때,
존재하던 데이터를 변경하는 게 아니라
새로운 데이터를 정의하여 반환한다.
이는 hook도 같다.
즉 예로 들어 배열 같은 경우 배열을 한 칸 추가할 때
push함수를 쓰면 에러가 뜬다.
하지만 createReducer에서는 push함수를 사용할 수 있다.
존재하던 데이터에서 수정하는 방식으로 사용 가능하단 것이다.
이는 지금 다루기엔 예제가 복잡해질까 봐 나눠서 설명하려 하는데,
일단 그러한 단순히 switch 문을 안 써도 되는 것에 끝나는 게 아니라,
그보다 더 중점인 장점도 있다는 것이다.
끝
으으음...
생각보다 양이 많은 것 같다. 왜지
일단 여기서 끊어야겠다.
더 적어봤자 읽는 사람도 효율이 떨어질 것 같다.
'웹개발(프론트) > React' 카테고리의 다른 글
createElement 스타일 변경 (0) | 2021.04.18 |
---|---|
styled components 심화편 (0) | 2021.04.17 |
Redux by react (0) | 2021.04.14 |
Redux (0) | 2021.04.12 |
useMemo (0) | 2021.04.04 |
- Total
- Today
- Yesterday
- nodejs
- Router
- proptype
- homebrew
- 비동기
- usecookies
- 랜더링
- Redux
- 클릭
- touchable
- switch
- useReducer
- react
- 쿠키
- CSS
- 웹접근성
- html
- 가상샐렉터
- 서버
- 에러
- 이쁜코드
- visualcode
- 무료아이콘
- 아이콘
- 접근성
- SVG
- async
- Hook
- Expo
- dom
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |