티스토리 뷰
... redux에 대해 또 쓸게 생겼다.
저번에 마지막이라고 하지를 말껄
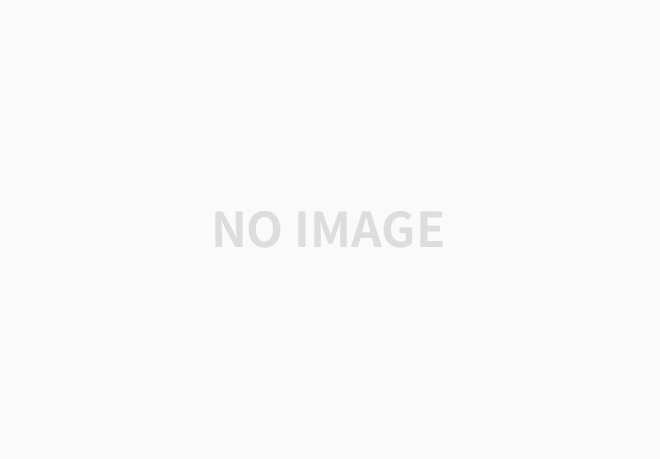
redux를 쓰면서 여러 가지 데이터를 한 번에 수정(dispatch)하려는데
그런 상황에 대한 예제가 없었다
다른 action을 각각 실행시켜도 되긴 하는데,
그렇게 하면 한번 dispatch 하는 것보다 비효율적인 것 같아
어떻게든 도전하다가 성공시켰다.
Redux 데이터 여러 개 수정하기
코드는 저번 포스트에서 응용할 것이다.
Redux-slice
목차 역시 학은 쉽고 습은 어렵다. 그래도 해결했으니 다행이다. "학(學)과 습(習)"의 차이를 아시나요? 오늘도 생뚱맞은 질문으로 포스팅을 시작해 보겠습니다. "학(學)과 습(習)"의 차이를 아시
sirong.tistory.com
//index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { Provider } from 'react-redux';
import store from './Store';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
//Store.js
import { configureStore, createSlice } from "@reduxjs/toolkit";
const store = createSlice({
name: "storeReducer",
initialState: {
text:'',
count: 0
},
reducers: {
add: (state, action) => {state.text = action.payload},
plus: (state, action) => {
return {
...state,
count: action.payload+1
}
}
}
});
export const { add, plus } = store.actions;
export default configureStore({ reducer: store.reducer });
//App.js
import React, { useState } from "react";
import { connect } from "react-redux";
import {add, plus} from './Store';
function App({store, addText, plusCount}) {
const [text,setText] = useState('');
function handleInput(e){
setText(e.target.value);
}
function onSubmit(e){
e.preventDefault();
addText(text);
setText('');
}
function onClick(e){
console.log(store.count);
plusCount(store.count);
}
return (
<>
{store.count}<button onClick={onClick}>Plus Count</button>
<form onSubmit={onSubmit}>
<input value={text} onChange={handleInput}/>
<button>Submit</button>
</form>
{store.text}
</>
);
}
function mapStateToProps(state) {
return { store: state };
}
function mapDispatchToProps(dispatch) {
return {
addText: text => dispatch(add(text)),
plusCount: (count) => dispatch(plus(count))
};
}
export default connect(mapStateToProps, mapDispatchToProps)(App);
코드
//Store.js
import { configureStore, createSlice } from "@reduxjs/toolkit";
const store = createSlice({
name: "storeReducer",
initialState: {
text:'',
count: 0
},
reducers: {
add: (state, action) => {state.text = action.payload},
plus: (state, action) => {
return {
count: action.payload.count,
text: action.payload.text
}
}
}
});
export const { add, plus } = store.actions;
export default configureStore({ reducer: store.reducer });
//App.js
import React, { useState } from "react";
import { connect } from "react-redux";
import {add, plus} from './Store';
function App({store, addText, plusCount}) {
const [text,setText] = useState('');
function handleInput(e){
setText(e.target.value);
}
function onSubmit(e){
e.preventDefault();
addText(text);
setText('');
}
function onClick(){
plusCount(store.count, text);
}
return (
<>
{store.count}<button onClick={onClick}>Plus Count</button>
<form onSubmit={onSubmit}>
<input value={text} onChange={handleInput}/>
<button>Submit</button>
</form>
{store.text}
</>
);
}
function mapStateToProps(state) {
return { store: state };
}
function mapDispatchToProps(dispatch) {
return {
addText: text => dispatch(add(text)),
plusCount: (count, text) => dispatch(plus({count, text}))
};
}
export default connect(mapStateToProps, mapDispatchToProps)(App);
해석
//App.js
function mapDispatchToProps(dispatch) {
return {
plusCount: (count, text) => dispatch(plus({count, text}))
};
}
dispatch plusCount 인자를 두 개로 늘리고,
dispatch로 전해지는 데이터도 두개로 늘려준다.
//App.js
function onClick(){
plusCount(store.count, text);
}
dispatch plusCount에 데이터 두 개를 넣어준다.
//Store.js
import { configureStore, createSlice } from "@reduxjs/toolkit";
const store = createSlice({
name: "storeReducer",
initialState: {
text:'',
count: 0
},
reducers: {
add: (state, action) => {state.text = action.payload},
plus: (state, action) => {
return {
count: action.payload.count,
text: action.payload.text
}
}
}
});
export const { add, plus } = store.actions;
export default configureStore({ reducer: store.reducer });
reducer의 해당 action plus에
dispatch로 받은 데이터를 어떻게 적용시킬지 지정해준다.
포인트
오늘 한 과정의 포인트는
//App.js
function mapDispatchToProps(dispatch) {
return {
plusCount: (count, text) => dispatch(plus({count, text}))
};
}
dispatch에 넣을 여러 데이터를
하나의 object로 묶는 것이다.
//Store.js
plus: (state, action) => {
return {
count: action.payload.count,
text: action.payload.text
}
}
dispatch에 개별의 데이터를 여러 개 넣으면 제일 앞에 하나만 payload로 전송된다.
때문에 하나인 payload가 여러 데이터를 가지도록 묶어주는 것이다.
끝
'웹개발(프론트)' 카테고리의 다른 글
정규표현식 (0) | 2021.04.30 |
---|---|
이쁜 코드를 짜는 규칙 (0) | 2021.04.29 |
Imported JSX component text must be in PascalCase or SCREAMING_SNAKE_CASE (0) | 2021.04.26 |
일러스트로 path 그리기 (0) | 2021.04.23 |
scrollto() (0) | 2021.04.22 |
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- visualcode
- 이쁜코드
- 에러
- 아이콘
- 접근성
- 랜더링
- async
- Router
- CSS
- useReducer
- usecookies
- proptype
- SVG
- dom
- Hook
- 가상샐렉터
- 비동기
- switch
- 서버
- nodejs
- homebrew
- 쿠키
- react
- Expo
- 무료아이콘
- 클릭
- touchable
- Redux
- 웹접근성
- html
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | |||||
3 | 4 | 5 | 6 | 7 | 8 | 9 |
10 | 11 | 12 | 13 | 14 | 15 | 16 |
17 | 18 | 19 | 20 | 21 | 22 | 23 |
24 | 25 | 26 | 27 | 28 | 29 | 30 |
31 |
글 보관함